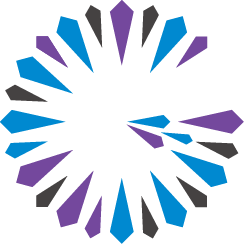
Pool Configuration Example and Settings
Connection pools require a standard client/server distributed system and cache configuration settings. You must also configure settings for the locator, server, and pool elements.
- Locator. Host and port where a server locator is listening.
- Server. Host and port where a server is listening.
- Pool. Client/server connection pool.
This page shows examples of creating a pool configuration programmatically using C++ code, and declaratively using XML.
Programmatic Pool Configuration
This example shows a programmatic pool configuration. For a list of the attributes you can configure, see PoolFactory
in the API docs.
cache.getPoolManager()
.createFactory()
.addLocator("localhost", 34756)
.setFreeConnectionTimeout(std::chrono::milliseconds(12345))
.setLoadConditioningInterval(std::chrono::milliseconds(23456))
.setMaxConnections(7)
.setMinConnections(3)
.setPingInterval(std::chrono::milliseconds(12345))
.setReadTimeout(std::chrono::milliseconds(23456))
.setRetryAttempts(3)
.setServerGroup("ServerGroup1")
.setSocketBufferSize(32768)
.setStatisticInterval(std::chrono::milliseconds(10123))
.setSubscriptionAckInterval(std::chrono::milliseconds(567))
.setSubscriptionEnabled(true)
.setSubscriptionMessageTrackingTimeout(std::chrono::milliseconds(900123))
.setSubscriptionRedundancy(0)
.setThreadLocalConnections(true)
.create("test_pool_1");
Declarative Pool Configuration
This example shows a declarative pool configuration. Following the example is a table that describes the XML pool attributes you can configure.
Note:
You create an instance of PoolFactory
through PoolManager
.
<pool free-connection-timeout="12345" idle-timeout="5555"
load-conditioning-interval="23456"
max-connections="7" min-connections="3"
name="test_pool_1" ping-interval="12345"
read-timeout="23456" retry-attempts="3" server-group="ServerGroup1"
socket-buffer-size="32768" statistic-interval="10123"
subscription-ack-interval="567" subscription-enabled="true"
subscription-message-tracking-timeout="900123"
subscription-redundancy="0" thread-local-connections="true">
<locator host="localhost" port="34756"/>
</pool>
Pool Attributes
Attribute Name | Description | Default |
---|---|---|
free-connection-timeout |
Number of milliseconds (ms) that the client waits for a free connection if |
10000 ms |
|
Number of milliseconds to wait for a connection to become idle for load balancing |
5000 ms |
load-conditioning-interval |
Interval in which the pool checks to see if a connection to a specific server should be moved to a different server to improve the load balance. |
300000 ms (5 minutes) |
max-connections |
Maximum number of connections that the pool can create. If all connections are in use, an operation requiring a client-to server-connection is blocked until a connection is available or the
Note:
If you use this setting to cap your pool connections, disable the pool attribute |
-1 |
|
Number of connections that must be created initially. |
5 |
|
Pool name. |
|
|
Interval between pinging the server to show the client is alive, set in milliseconds. Pings are only sent when the |
10000 ms |
|
Setting used for single-hop access to partitioned region data in the servers for some data operations. See PartitionResolver. See note in |
True |
|
Number of milliseconds to wait for a response from a server before the connection times out. |
10000 |
|
Number of times to retry an operation after a time-out or exception for high availability. If set to -1, the pool tries every available server once until it succeeds or has tried all servers. |
-1 |
|
Server group from which to select connections. If not specified, the global group of all connected servers is used. |
empty |
|
Size of the socket buffer, in bytes, on each connection established. |
32768 |
|
Default frequency, in milliseconds, with which the client statistics are sent to the server. A value of |
-1 |
|
Number of milliseconds to wait before sending an acknowledgment to the server about events received from the subscriptions. |
100 |
|
Whether to establish a server to client subscription. |
False |
|
Number of milliseconds for which messages sent from a server to a client are tracked. The tracking is done to minimize duplicate events. |
90000 |
|
Redundancy for servers that contain subscriptions established by the client. A value of |
0 |
|
Whether the connections must have affinity to the thread that last used them.
Note:
To set this to |
False |
update-locator-list-interval |
An integer number of milliseconds defining the interval between locator list updates. If the value is less than or equal to 0, the update will be disabled. | 5000 |