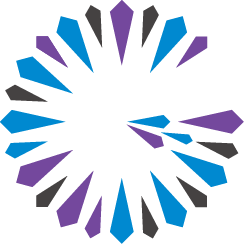
Remote Queries
Use the remote query API to query your cached data stored on a cache server.
Remote Query Basics
Queries are evaluated and executed on the cache server, and the results are returned to the client. You can optimize your queries by defining indexes on the cache server.
Querying and indexing operate only on remote cache server contents.
Note: Because floating point numbers can not reliably be compared for equality, do not use floating point values as keys or key components when constructing a query.
Query language: OQL
Geode provides a SQL-like querying language called OQL that allows you to access data stored in Geode regions. OQL is very similar to SQL, but OQL allows you to query complex objects, object attributes, and methods.
In the context of a query, specify the name of a region by its full path, starting with a slash (/
).
The query language supports drilling down into nested object structures. Nested data collections can be explicitly referenced in the FROM clause of a query.
A query execution returns its results as either a ResultSet
or a StructSet
.
Query language features and grammar are described in the Geode manual at Querying.
Creating Indexes
Indexes can provide significant performance gains for query execution. You create and maintain indexes on the cache server. For detailed information about working with indexes configured on a cache server, see Working with Indexes in the server’s documentation.
Remote Query API
This section gives a general overview of the interfaces and classes that are provided by the query package API.
Query
You must create a Query
object for each new query. The Query
interface provides methods for
managing the compilation and execution of queries, and for retrieving an existing query string.
A Query
is obtained from a QueryService
, which is obtained from one of two sources:
To create a
Query
that operates on the Geode server, useapache::geode::client::Pool::getQueryService()
to instantiate aQueryService
obtained from aPool
.To create a
Query
that operates on your application’s local cache, useapache::geode::client::Cache::getQueryService()
to instantiate aQueryService
obtained from aCache
.
Executing a Query from the Client
The essential steps to create and execute a query are:
- Create an instance of the
QueryService
class. If you are using the pool API (recommended), you should obtain theQueryService
from the pool. - Create a
Query
instance that is compatible with the OQL specification. - Use the
Query.execute()
method to submit the query string to the cache server. The server remotely evaluates the query string and returns the results to the client. - Iterate through the returned objects.
C++ Query Example
These C++ code excerpts are from the examples/cpp/remotequery
example included in your client
distribution. See the example for full context.
Following the steps listed above,
Obtain a
QueryService
object from the connection pool:std::shared_ptr<QueryService> queryService = nullptr; queryService = pool->getQueryService();
Create a
Query
object by callingQueryService.newQuery()
, specifying your OQL query as a string parameter:auto query = queryService->newQuery("SELECT * FROM /custom_orders WHERE quantity > 30");
Execute the query. Collect the query output, returned as either a
ResultSet
or aStructSet
, and iterate through the results:auto queryResults = query->execute(); for (auto&& value : *queryResults) { auto&& order = std::dynamic_pointer_cast<Order>(value); std::cout << order->toString() << std::endl; }