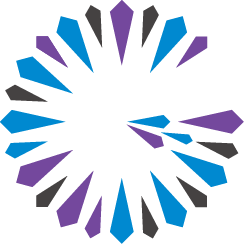
Authentication
A client is authenticated when it connects with valid credentials to a Geode cache server that is configured with the client authentication callback. For details on the server’s role in authentication and what it expects from the client, see Implementing Authentication in the Geode User Guide.
In your application, authentication credentials must be set when creating the cache. In practice, this means setting the authentication credentials when you create the CacheFactory.
.NET Framework Authentication Example
In this C# authentication example, the CacheFactory
creation process sets the authentication callback:
var cache = new CacheFactory()
.Set("log-level", "none")
.SetAuthInitialize(new ExampleAuthInitialize())
.Create();
Credentials are implemented in the GetCredentials
member function of the ExampleAuthInitialize
class, which implements the IAuthInitialize
interface:
class ExampleAuthInitialize : IAuthInitialize
{
public ExampleAuthInitialize()
{
// TODO initialize your resources here
Console.Out.WriteLine("ExampleAuthInitialize::ExampleAuthInitialize called");
}
public void Close()
{
// TODO close your resources here
Console.Out.WriteLine("ExampleAuthInitialize::Close called");
}
public Properties<string, object> GetCredentials(Properties<string, string> props, string server)
{
// TODO get your username and password
Console.Out.WriteLine("ExampleAuthInitialize::GetCredentials called");
var credentials = new Properties<string, object>();
credentials.Insert("security-username", "root");
credentials.Insert("security-password", "root");
return credentials;
}
}