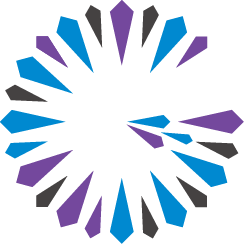
Continuous Queries
The C++ and .NET clients can initiate queries that run on the Geode cache server and notify the client when the query results have changed. For details on the server-side setup for continuous queries, see How Continuous Querying Works in the Geode User Guide.
Continuous Query Basics
Continuous querying provides the following features:
Standard Geode native client query syntax and semantics. Continuous queries are expressed in the same language used for other native client queries. See Remote Queries.
Standard Geode events-based management of CQ events. The event handling used to process CQ events is based on the standard Geode event handling framework.
Complete integration with the client/server architecture. CQ functionality uses existing server-to-client messaging mechanisms to send events. All tuning of your server-to-client messaging also tunes the messaging of your CQ events. If your system is configured for high availability then your CQs are highly available, with seamless failover provided in case of server failure (see Highly Available Client/Server Event Messaging). If your clients are durable, you can also define any of your CQs as durable (see Implementing Durable Client/Server Messaging).
Interest criteria based on data values. Continuous queries are run against the region’s entry values. Compare this to register interest by reviewing Registering Interest for Entries.
Active query execution. Once initialized, the queries operate on new events. Events that change the query result are sent to the client immediately.
Note: Because floating point numbers can not reliably be compared for equality, do not use floating point values as keys or key components when constructing a query.
Typical Continuous Query Lifecycle
- The client creates the CQ. This sets up everything for running the query and provides the client with a
CqQuery
object, but does not execute the CQ. At this point, the query is in aSTOPPED
state, ready to be closed or run. The client initiates the CQ with an API call to one of the
CqQuery Execute*
methods. This puts the query into aRUNNING
state on the client and on the server. The server remotely evaluates the query string, and optionally returns the results to the client.CqQuery Execute*
methods include:CqQuery.Execute()
CqQuery.ExecuteWithInitialResults()
A CQ Listener waits for events. When it receives events, it takes action accordingly with the data in the CqEvent.
The CQ is closed by a client call to
CqQuery.Close
. This de-allocates all resources in use for the CQ on the client and server. At this point, the cycle could begin again with the creation of a newCqQuery
instance.
Executing a Continuous Query from the Client
The essential steps to create and execute a continuous query are:
- Create an instance of the
QueryService
class. If you are using the pool API (recommended), you should obtain theQueryService
from the pool. - Define a CQ Listener (an
ICqListener
) to field events sent from the server. - Use one of the
CqQuery Execute*
methods to submit the query string to the cache server. - The server remotely evaluates the query string, then monitors those results and notifies the client if they change.
- The client listens for changes that match the query predicate.
- Iterate through the returned objects.
- When finished, close down the continuous query.
.NET Continuous Query Example
These C# code excerpts are from the examples\dotnet\continuousquery
example included in your client
distribution. See the example for full context.
Following the steps listed above,
Create a query service:
var queryService = pool.GetQueryService();
Define an ICqListener:
public class MyCqListener<TKey, TResult> : ICqListener<TKey, TResult> {
Create an instance of your ICqListener and insert it into a CQ attributes object:
var cqListener = new MyCqListener<string, Order>(); var cqAttributesFactory = new CqAttributesFactory<string, Order>(); cqAttributesFactory.AddCqListener(cqListener); var cqAttributes = cqAttributesFactory.Create();
Create a Continuous Query using the query service and the CQ attributes:
var query = queryService.NewCq("MyCq", "SELECT * FROM /example_orderobject WHERE quantity > 30", cqAttributes, false);
Execute the query:
query.Execute();
Wait for events and do something with them.
/* Excerpt from the CqListener */ /* Determine Operation Type */ switch (ev.getQueryOperation()) { case CqOperation.OP_TYPE_CREATE: operationType = "CREATE"; break; case CqOperation.OP_TYPE_UPDATE: operationType = "UPDATE"; break; case CqOperation.OP_TYPE_DESTROY: operationType = "DESTROY"; break; default: break; } ... /* Take action based on OP Type */
When finished, close up shop.
query.Execute(); ... (respond to events as they arrive) query.Stop(); query.Close(); cache.Close();