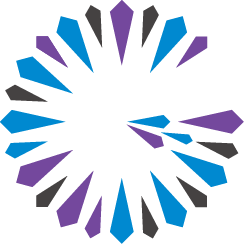
How to Safely Modify the Cache from an Event Handler Callback
Event handlers are synchronous. If you need to change the cache or perform any other distributed operation from event handler callbacks, be careful to avoid activities that might block and affect your overall system performance.
Operations to Avoid in Event Handlers
Do not perform distributed operations of any kind directly from your event handler. Geode is a highly distributed system and many operations that may seem local invoke distributed operations.
These are common distributed operations that can get you into trouble:
- Calling
Region
methods, on the event’s region or any other region. - Using the Geode
DistributedLockService
. - Modifying region attributes.
- Executing a function through the Geode
FunctionService
.
To be on the safe side, do not make any calls to the Geode API directly from your event handler. Make all Geode API calls from within a separate thread or executor.
How to Perform Distributed Operations Based on Events
If you need to use the Geode API from your handlers, make your work asynchronous to the event handler. You can spawn a separate thread or use a solution like the java.util.concurrent.Executor
interface.
This example shows a serial executor where the callback creates a Runnable
that can be pulled off a queue and run by another object. This preserves the ordering of events.
public void afterCreate(EntryEvent event) {
final Region otherRegion = cache.getRegion("/otherRegion");
final Object key = event.getKey();
final Object val = event.getNewValue();
serialExecutor.execute(new Runnable() {
public void run() {
try {
otherRegion.create(key, val);
}
catch (org.apache.geode.cache.RegionDestroyedException e) {
...
}
catch (org.apache.geode.cache.EntryExistsException e) {
...
}
}
});
}
For additional information on the Executor
, see the SerialExecutor
example on the Oracle Java web site.