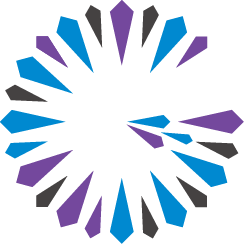
Writing and Executing a Query in OQL
The Geode QueryService provides methods to create the Query object. You can then use the Query object to perform query-related operations.
The QueryService instance you should use depends on whether you are querying the local cache of an application or if you want your application to query the server cache.
Querying a Local Cache
To query the application’s local cache or to query other members, use org.apache.geode.cache.Cache.getQueryService
.
Sample Code
// Identify your query string.
String queryString = "SELECT DISTINCT * FROM /exampleRegion";
// Get QueryService from Cache.
QueryService queryService = cache.getQueryService();
// Create the Query Object.
Query query = queryService.newQuery(queryString);
// Execute Query locally. Returns results set.
SelectResults results = (SelectResults)query.execute();
// Find the Size of the ResultSet.
int size = results.size();
// Iterate through your ResultSet.
Portfolio p = (Portfolio)results.iterator().next(); /* Region containing Portfolio object. */
Querying a Server Cache from a Client
To perform a client to server query, use org.apache.geode.cache.client.Pool.getQueryService
.
Sample Code
// Identify your query string.
String queryString = "SELECT DISTINCT * FROM /exampleRegion";
// Get QueryService from client pool.
QueryService queryService = pool.getQueryService();
// Create the Query Object.
Query query = queryService.newQuery(queryString);
// Execute Query locally. Returns results set.
SelectResults results = (SelectResults)query.execute();
// Find the Size of the ResultSet.
int size = results.size();
// Iterate through your ResultSet.
Portfolio p = (Portfolio)results.iterator().next(); /* Region containing Portfolio object. */
Refer to the following JavaDocs for specific APIs:
Note:
You can also perform queries using the gfsh query
command. See query.
Next Steps
To build a query string, you combine supported keywords, expressions, and operators to create an expression that returns the information you require.
This section covers the following querying language features.
This section describes some limitations of which developers should be aware when composing queries in OQL.