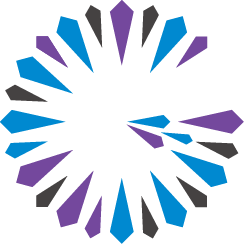
Managing Data Entries
Program your applications to create, modify, and manage your cached data entries.
Note:
If you do not have the cache’s copy-on-read
attribute set to true, do not change the objects returned from the Java entry access methods. See Safe Entry Modification.
Keys
Geode calls hashCode()
on the key
to map an entry within the region.
The hashCode()
return value must be the same for
a given key on every server that hosts the region.
An equals()
call return value on a given key also must be
the same on every server that hosts the region.
A key may be a primitive type or a custom class. For custom classes, see Classes Used as Keys.
Do not use an enumerated type (enum
) for a key.
The enum
hashCode()
may not be overridden,
and its hash code is based upon an address.
Therefore, the return value for a hashCode()
call can be different
on each server, violating the restriction that it must return
the same value on every server that hosts the region.
Basic Create and Update
To create or update an entry in the cache, use Region.put
. For example:
String name = ...
String value = ...
this.currRegion.put(name,value);
Note:
You can also use the gfsh put
command to add entries to a region, and the get
command to retrieve entries from a region. See get and put for more information.
If you want only to create the entry (with a null value and with method failure if the entry already exists), use Region.create
instead.
Batch Operations (getAll, putAll, removeAll)
Geode provides three APIs to perform batch operations on multiple region entries:
-
Region.getAll
-
Region.putAll
-
Region.removeAll
The getAll
method takes a collection of keys and returns a Map
of values for the provided keys. If a given key does not exist in the region, then that key’s value in the returned map will be null.
The putAll
method takes a Map
of key-value pairs and puts them into the cache and distributes them in a single operation.
Example:
void putAll(String command) throws CacheException
{
// Get Entry keys and values into Strings key1, ... keyN and value1, ... valueN
Map map = new LinkedHashMap();
map.put(key1, value1));
...
map.put(keyN, valueN));
this.currRegion.putAll(map);
}
The updates to the cache are done individually in the order in which they were placed in the Map
. For partitioned regions, multiple events are sent as a single message to the primary buckets and then distributed to the secondary buckets.
Note: The processing of maps with very many entries and/or very large data may affect system performance and cause cache update timeouts, especially if the region uses overflow or persistence to disk.
The removeAll
method takes a collection of keys and removes all of the entries for the specified keys from this region. This call performs the equivalent of callingdestroy(Object)
on this region once for each key in the specified collection. If an entry does not exist, then that key is skipped. An EntryNotFoundException
is not thrown. This operation will be distributed to other caches if the region’s scope is not set to Scope.LOCAL
.
Safe Entry Modification
When you get an entry value from the cache, by default, the retrieval methods return a direct reference to the cached object. This provides the value as quickly as possible, but also opens the cache to direct, in-place changes.
Note: Do not directly modify cached values. Modifying a value in place bypasses the Geode distribution framework, including cache writers and listeners, expiration activities, and transaction management, and can produce undesired results.
Always change your entries using copies of the retrieved objects—never directly modify the returned objects. You can do this in one of two ways:
Change the entry retrieval behavior for your cache by setting the cache attribute,
copy-on-read
, to true (the default is false).<cache copy-on-read="true"> ... </cache>
When
copy-on-read
is true, the entry access methods return copies of the entries. This protects you from inadvertently modifying in-place, but negatively impacts performance and memory consumption when copying is not needed.These entry access methods return an entry reference if
copy-on-read
is false and a copy of the entry ifcopy-on-read
is true:Region.get
result ofRegion.put
EntryEvent.getNewValue
Region.values
Region.Entry.getValue
EntryEvent.getOldValue
Query.select
Create a copy of the returned object and work with that. For objects that are cloneable or serializable, you can copy the entry value to a new object using
org.apache.geode.CopyHelper.copy
. Example:Object o = (StringBuffer)region.get("stringBuf"); StringBuffer s = (StringBuffer) CopyHelper.copy(o); s.append("Changes to value, added using put."); region.put("stringBuf", s);
Retrieving Region Entries from Proxy Members
The Region.values
method call applies to the local region instance only. If you call the values
method from a client region using the PROXY shortcut, the method call will not be redirected to the server region. To obtain a collection of all values in the Region from a client, you should use interest registration on ALL_KEYS, or use a query.
If you use the Region.get
method from a proxy member, the method call will redirect to the region on the server if it cannot find the key locally.
Using gfsh to get and put
You can use the gfsh get
and put
commands to manage data. See get and put.
For example:
get --key=('id':'133abg124') --region=region1
// Retrieving when key type is a wrapper(primitive)/String
get --key=('133abg124') --region=/region1/region12 --value-class=data.ProfileDetails
get --key=('100L') --region=/region1/region12 --value-class=data.ProfileDetails
--key-class=java.lang.Long
put --key=('id':'133abg125') --value=('firstname':'James','lastname':'Gosling')
--region=/region1 --key-class=data.ProfileKey --value-class=data.ProfileDetails
put --key=('133abg124') --value=('Hello World!!') --region=/region2
put --key=('100F') --value=('2146547689879658564') --region=/region1/region12
--key-class=java.lang.Float --value-class=java.lang.Long