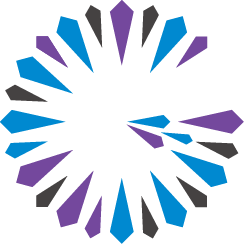
Customizing Serialization with Class Pattern Strings
Use class pattern strings to name the classes that you want to serialize using Geode’s reflection-based autoserializer and to specify object identity fields and to specify fields to exclude from serialization.
The class pattern strings used to configured the ReflectionBasedAutoSerializer
are standard regular expressions. For example, this expression would select all classes defined in the com.company.domain
package and its subpackages:
com\.company\.domain\..*
You can augment the pattern strings with a special notation to define fields to exclude from serialization and to define fields to mark as PDX identity fields. The full syntax of the pattern string is:
<class pattern> [# (identity|exclude) = <field pattern>]... [, <class pattern>...]
The following example pattern string sets these PDX serialization criteria:
- Classes with names matching the pattern
com.company.DomainObject.*
are serialized. In those classes, fields beginning withid
are marked as identity fields and fields namedcreationDate
are not serialized. - The class
com.company.special.Patient
is serialized. In the class, the field,ssn
is marked as an identity field
com.company.DomainObject.*#identity=id.*#exclude=creationDate,
com.company.special.Patient#identity=ssn
Note:
There is no association between the identity
and exclude
options, so the pattern above could also be expressed as:
com.company.DomainObject.*#identity=id.*, com.company.DomainObject.*#exclude=creationDate,
com.company.special.Patient#identity=ssn
Note: The order of the patterns is not relevant. All defined class patterns are used when determining whether a field should be considered as an identity field or should be excluded.
Examples:
This XML uses the example pattern shown above:
<parameter name="classes"> <string>com.company.DomainObject.*#identity=id.*#exclude=creationDate, com.company.special.Patient#identity=ssn</string> </parameter>
This application code sets the same pattern:
classPatterns.add("com.company.DomainObject.*#identity=id.*#exclude=creationDate, com.company.special.Patient#identity=ssn");
This application code has the same effect:
Cache c = new CacheFactory().set("cache-xml-file", cacheXmlFileName) .setPdxSerializer(new ReflectionBasedAutoSerializer("com.foo.DomainObject*#identity=id.*", "com.company.DomainObject.*#exclude=creationDate","com.company.special.Patient#identity=ssn")) .create();